Example with Next.js
An example with Next.js is available in this dedicated repository as in this repository folder.
There are a couple of configuration and requirement to run Jupyter React in Next.js.
Yarn V3
You have to build with Yarn V3 (Npm or Pnpm do not work for now).
Render in client-mode
You need to render in client-mode the Jupyter part.
To achieve this, you can e.g. load your page with external components dynamically loaded.
import dynamic from 'next/dynamic'
const HomePageNoSSR = dynamic(
() => import('../components/HomeComponent'),
{ ssr: false }
);
function Home() {
return (
<>
<HomePageNoSSR />
</>
)
}
export default Home
The components should ideally reside outside of the app
folder. Add 'use client'
at the top of your React.js components.
'use client'
import { Jupyter, Notebook, CellSidebar } from '@datalayer/jupyter-react';
// ...
Add Require.js
For IPyWidgets support, you need requirejs
on the page, so add a script
tag to your layout to load the script from a CDN https://cdnjs.cloudflare.com/ajax/libs/require.js/2.3.4/require.min.js
.
import './globals.css'
import Script from 'next/script'
import { Inter } from 'next/font/google'
const inter = Inter({ subsets: ['latin'] })
export const metadata = {
title: 'Create Next App',
description: 'Generated by create next app',
}
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en">
<script src="https://cdnjs.cloudflare.com/ajax/libs/require.js/2.3.4/require.min.js" />
<body className={inter.className}>{children}</body>
</html>
)
}
CSS
We have seen that Jupyter React components places in the main tag of the default Next.js application are not shown (they have a width of 0).
For now, please place you components outside of the main Next.js CSS.
Enjoy!
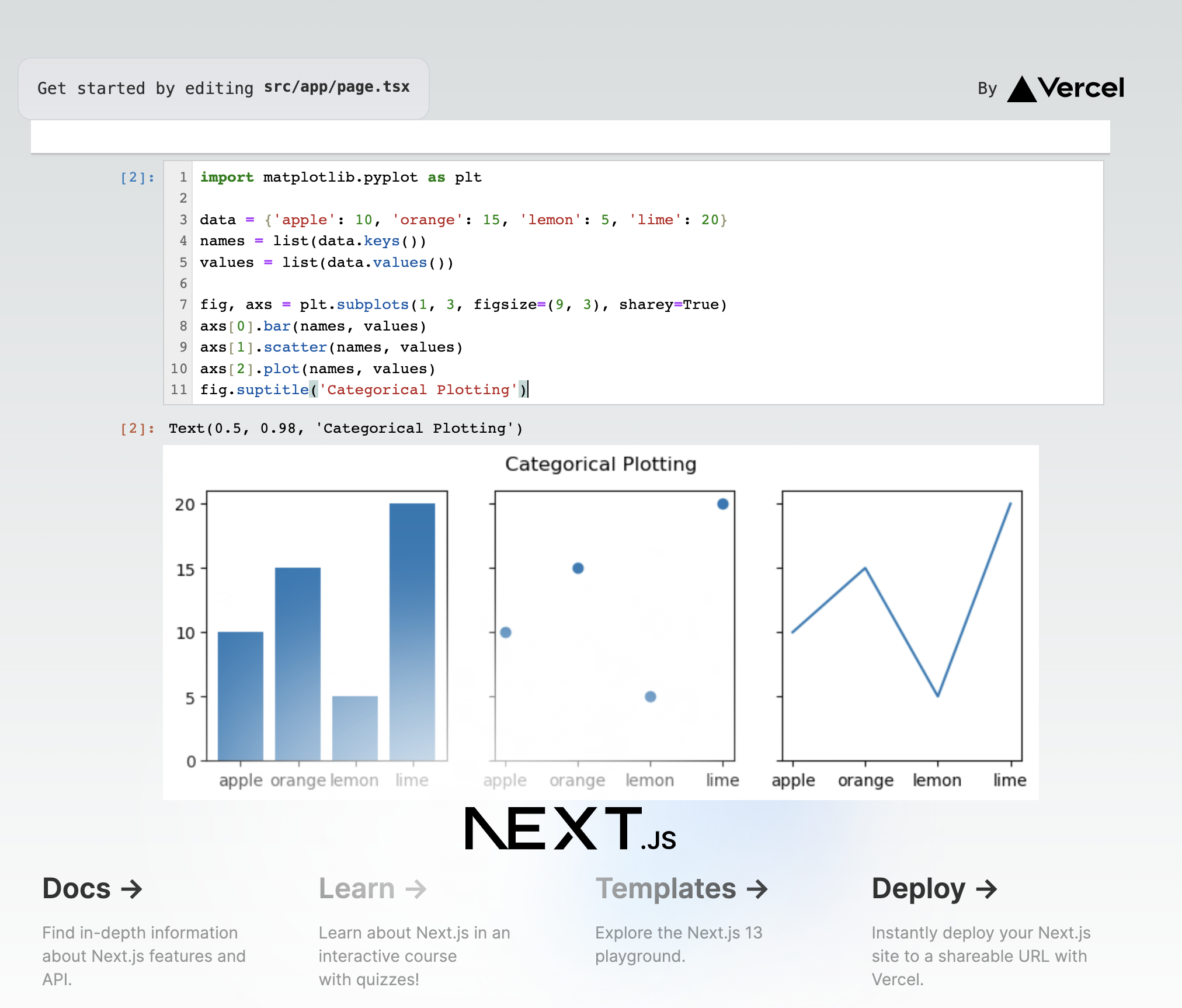